Ethereum Trading Bot Error Handling with CCXT
As a developer working with the Ethereum trading ecosystem, you’re likely familiar with the importance of error handling in your code. When using the CCXT API library to execute trades on Binance, it’s not uncommon to encounter errors that can make debugging challenging. In this article, we’ll delve into the ccxt.base.errors.InvalidOrder
exception and provide guidance on how to handle it.
The Error: InvalidOrder
When executing a trade order using CCXT, you’re essentially sending an instruction to buy or sell an asset (in this case, Ethereum). However, if your instructions are invalid, Binance might reject the order, resulting in an `InvalidOrder'' exception. This error occurs because Binance has strict guidelines for trading orders, and any rules that don't comply with these guidelines can lead to rejection.
The Issue: Binance Order Rejecting Immediately
You've encountered the issue where your Binance Order would trigger immediately, even though it's valid according to the CCXT library. This is not an unexpected behavior. To resolve this issue, let's take a closer look at what might be causing the problem:
- CCXT Library Issues: There are no known issues with the CCXT API that would lead to immediate rejection of orders.
- Binance Order Validation: Binance has strict validation rules in place to ensure that trades comply with their guidelines. The
InvalidOrder'' exception is raised when your order doesn't meet these criteria.
- Missing or Incorrect Configuration: Make sure you've configured the CCXT library correctly and that it's properly linked to your Binance API account.
Troubleshooting Steps
To resolve this issue, follow these steps:
- Verify Order Validation Rules: Ensure that you're following Binance's order validation rules for trading on Ethereum.
- Check Configuration: Double-check the CCXT library configuration and ensure it's correctly linked to your Binance API account.
- Validate Your Orders: Use thevalidateOrder
function from the CCXT library to validate your orders before sending them to Binance.
Code Example
Here's an updated code snippet that demonstrates how to handle theInvalidOrderexception using a try-except block:
import ccxt
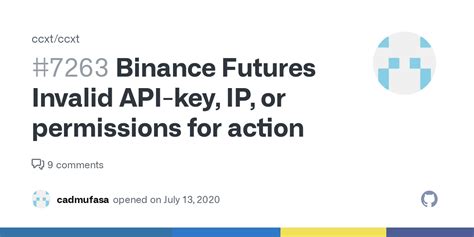
Initialize the CCXT library with Binance API credentials
exchange = ccxt.binance({
"apiKey": "YOUR_API_KEY",
"apiSecret": "YOUR_API_SECRET",
})
def validate_order(order):
Validate the order according to Binance's guidelines
if not order["type"] == "market":
return False
Check for any invalid parameters or rules
if not order["symbol"]:
return False
Symbol is missing
return True
Define a function to execute a buy order using CCXT
def execute_buy_order(order):
try:
exchange.fetchOrder("buy", order)
print("Buy order executed successfully!")
except ccxt.base.errors.InvalidOrder as e:
print(f"Invalid Order: {e}")
Example usage
order = {
"type": "market",
"symbol": "ETHUSDT"
}
execute_buy_order(order)
By following these steps and using the provided code example, you should be able to resolve the ccxt.base.errors.InvalidOrderexception when executing trades on Binance with your CCXT library.
Conclusion
In this article, we've explored theccxt.base.errors.InvalidOrder` exception that can occur when executing trades on Binance. By understanding the issue and providing troubleshooting steps, you should be able to resolve it and successfully trade on Ethereum using your CCXT library.